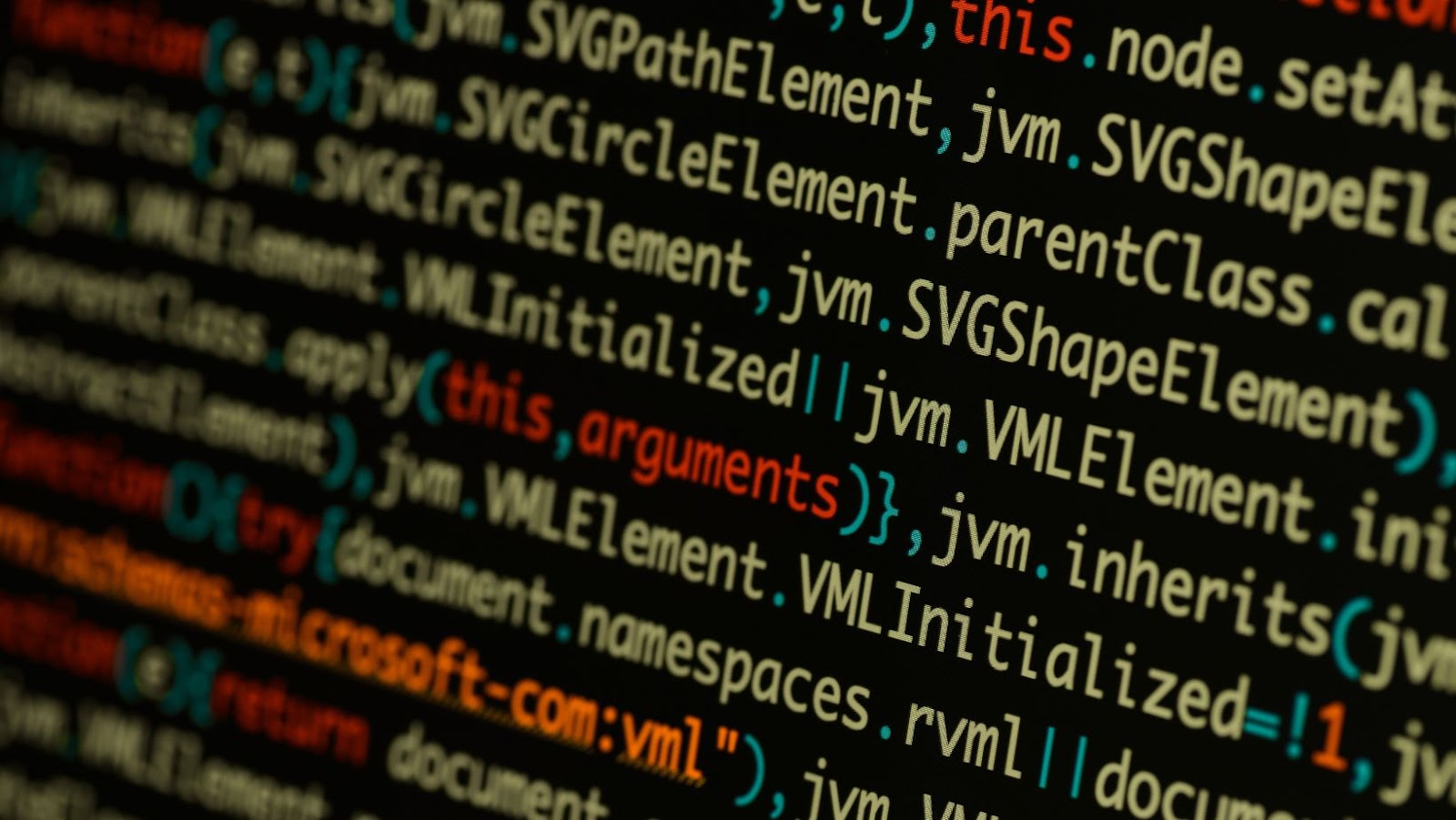
Upcasting in Java is the process of converting a child class to its parent class. This is done to simplify interactions between different parts of the program or to take advantage of any features a parent class may have. Java makes it easy to upcast objects, and doing so can help you write cleaner code.
In this article, we’ll discuss the details and give some examples of upcasting in Java.
What is upcasting in java
Upcasting is a type of polymorphism in the Java programming language. Polymorphism allows an object to take on multiple types at runtime, and upcasting is the process of changing an object’s type to one of its superclasses or implemented interfaces. Upcasting is sometimes called widening, because in doing it you are widening the scope of what types you can refer to the object as.
Upcasting allows a child class object to be treated as if it were a parent class object, and makes it possible for multiple objects from different types to be used interchangeably in programming applications. Doing so means you don’t have to create specialized references for each and every subclass; rather, you can relate all subclasses and treat them as if they were all members of a single type – their common parent type.
It’s important to note that when upcasted, all methods associated with the original (child) type are hidden, so that only methods associated with the new (parent)type remain (i.e. an object originally referred to as a ChildClass will no longer retain any methods specific for ChildClass; instead only method associated with ParentClass will exist). It’s like giving an object multiple hats: after upcasted it may no longer have access to certain methods – it’ll have different capabilities than with its original hat on!
Why Is Upcasting Used?
Upcasting is a programming technique applied in object-oriented languages like Java, C#, and C++. It allows a derived class to be typecasted as one of its parent classes. This is useful in cases where we want to access the available methods and features of a parent class while having an instance of the derived class. In other words, upcasting allows us to assign an instance of a derived (child) class to an instance of its parent (base) class.
Upcasting is particularly useful when you want to access multiple objects through the same interface or when you are trying to create more expressive generic code that can use multiple classes. This can often simplify coding tasks and allow for more flexibility when working with objects from different classes. Upcasting also allows for reusability of code as objects from different classes can be treated in the same way even if there are differences between them. Additionally, it makes it possible for derived types to receive less specialized treatment than the original type; i.e., subclasses can become superclasses via upcasting, but not vice versa – making it possible for developers to perform processing on a general level without having to consider details from specific subclasses.
How Does Upcasting Work?
Upcasting is a process used in Java that allows a variable of a subclass to be cast to its superclass. This allows a programmer to refer to an instance of a subclass using a variable of the superclass type. This can be a useful technique to write more concise and efficient code, as well as provide a means of abstraction.
Let’s look at how upcasting works in more detail.
Subclass to Superclass (Parent Class)
Upcasting in Java is the process of casting a reference from a subclass to its parent class. This allows the subclass object to access members of the parent class that are declared as protected or public.
It is also known as upcasting due to the fact that upcasting means casting an object to one of its ancestor classes in the object hierarchy.
Java supports two types of upcasting, implicit and explicit (or manual) upcasting. In implicit upcasting, there is no need for special syntax as Java automatically Typecasts an instance from a subclass to its parent class store in a reference variable with its appropriate name. For example, if you have an instance X from Class-B which extends from Class-A; then you can explicitly type cast it using the super keyword:
X = (Class-A) Y; // Here Y is instance of Class-B
With explicit or manual type casting, all member variables and methods exclusive to the child class will not be accessible when accessed through a reference variable of the parent or superclass type. The child’s exclusive methods and variables would still be accessible if accessed directly through an instance stored in a reference with type same as that of child or sub class type.
Upcasting also enables us to use methods belonging to different classes within method calls, which is helpful when dealing with inheritance tree structures (parent and children) with multiple layers and complexities. UpCasting can also improve code readability since it eliminates multiple casts when dealing with objects within many layers over multiple inheritance trees.
Subclass to Interface
Java, like other object-oriented languages, supports upcasting, meaning a variable of a base type such as an interface or class can be cast to another type that conforms to its criteria. For example, a reference of type Object can be cast to an instance of class Animal and then to an interface Mammal. Upcasting is often used for coercing polymorphism and function composition because it allows extended data or methods normally hidden from the source elements.
In the case of upcasting from subclass to interface, the referenced instance must implement all the methods defined on that interface in order for any changes made by those methods to be visible during runtime. The advantage of this approach is that it decouples type information from implementation details. This makes code easier to maintain and debug while still providing the ability to deliberately make changes when needed.
If you are new to writing Java code, you may find upcasting more intuitive than downcasting which requires casting an instance of an interface or class down its inheritance hierarchy instead.
Examples of Upcasting in Java
Upcasting is a powerful feature of the Java programming language. It is the process of converting a subtype to its super type. It is also referred to as implicit conversion. Upcasting is a useful tool when dealing with object-oriented programming as it allows you to write more concise and efficient code.
In this article, we will take a look at some examples of upcasting in Java:
Upcasting from Subclass to Superclass
When a subclass reference is assigned to a superclass reference, this process is called upcasting. In other words, when an object of the subclass is referred to by the superclass or parent class name, it is upcasting. This delegation from the subclass to the superclass is called upcasting.
Suppose we have two classes, Vehicle and Car. Here Vehicle is a superclass, and Car is its subclass. We will create an instance of Car and assign this instance to Vehicle type variable v:
Vehicle v = new Car(); //Upcasting
In this example, we are assigning an object of type Car to an object of type Vehicle. This process results in v referring to an object of the Car class referred as vehicle references i.e., a car referred as vehicle type. The upcasted code works similarly as if we had written write:
Car c = new Car(); //No Upcast
The benefit that you get from using upcasted code is that you can now refer to the object using both vehicle references and car references as you please (as long as it fits into what methods are available for use). In other words, upcasting allows you to call methods available in both classes without having to create multiple objects or extra coding for different types of objects – saving time and effort in data management tasks with multiple objects of different classes.
Upcasting from Subclass to Interface
Upcasting from subclass to interface is a type of casting which allows an object of a specific subclass to be referred to as it its common parent type, which in Java is typically an interface. Using this technique, the programmer can guarantee that regardless of the child class being used, certain methods and variables are available. This permits more easily extensible and re-usable code since changes to the child class will keep with the prescribed parent interface.
To illustrate, consider a class called “Shape“. This shape could have several child classes such as triangle, square and rectangle. Each child class may have unique variables or methods; however they still share many common elements such as “color” or “getArea()” that makes sense for all shapes.
By upcasting from subclass to interface in Java, we can refer to those common elements without bothering about the exact type we are working with – for example you can call “shapeObject.color” instead of “triangleObject.color” or “squareObject.color” or rely on “shapeObject.getArea()” instead of creating separate getArea() methods for every object type in our program.. As long as each individual shape implements the same interface (and thus implements getArea() and defines color) this upcast ensures that all those shared elements stay available across all objects without needing duplication of effort on part of programmer.
Advantages and Disadvantages of Upcasting
Upcasting in Java can be a helpful way to access data and functions from a parent class in a child class. It enables us to write more modular code by consolidating code and utilizing inheritance. However, there are certain drawbacks to upcasting in Java as well.
In this article, we will discuss the advantages and disadvantages of using upcasting in Java:
- Advantages of upcasting in Java
- Disadvantages of upcasting in Java
Advantages
Upcasting is when you assign a reference of a derived class object to a reference of one of its parent classes. This process allows for greater code reuse as non-related classes can be treated the same way. It also allows for safer code as it can help to prevent access to methods or variables that should remain private. As such, there are several advantages to upcasting in Java, which include:
- Achieving polymorphism – Upcasting aids in achieving polymorphism by assigning the same type of object through parents and children classes, allowing them to be treated the same way regardless of their actual type.
- Improved Code Reuse – By casting a child class up to its parent type, multiple instances of an unrelated child class can be operated on without risk or redundancy since they would have the same parent type.
- Simplified Code – Utilizing generic methods, objects created from unrelated classes can often operate on their individual data types without needing redundant code.
- Enforced Encapsulation – By limiting access at compile time with the aid of upcasting , projects using inheritance achieve improved security and integrity as values will not get mistakenly called from outside interfaces or objects that should not accessed .
Disadvantages
Upcasting has some disadvantages as well. Since upcasted references are not type-checked, they can refer to objects that do not have the appropriate behavior set. This reduces the flexibility of downcasting as compared to explicit casting as it prevents compile-time type checking. Therefore, when using upcasting with unchecked exceptions, one has to be wary of potential problems at runtime that otherwise could have been avoided at compile time when using explicit casting.
Another drawback of upcasting is the introduction of unnecessary clutter in code and a decrease in code readability if used heavily. In frequent scenarios, an object reference needs to be cast multiple times from a subclass type all the way to its superclass ancestor. This makes it hard for users unfamiliar with Java’s casting system to immediately understand what is being done within the code base.
Additionally, there is a limitation on how far upcasted references can go in Java; one cannot go beyond the Object class even though its methods may no longer be applicable depending on the ancestor’s real type or its context in a particular situation. As an example, if one attempted to invoke a method on an Object reference pointing only at an instance variable containing a String (“Foo”) Java would report that “[Object] doesn’t contain method invoke()” at runtime even though invoking this method would not make sense due to this reference being out of context to operate correctly anyway.
Conclusion
In conclusion, upcasting is a process used by the Java compiler to convert a type within a hierarchy of types from a lower-level type to a higher-level type. Upcasting is beneficial in that it allows developers to assign objects of an unknown type to an object of one of its super classes, thus simplifying interactions and making reuse easier.
Although upcasting can lead to reduced performance if not used judiciously, it is an important tool in the Java developer’s toolbox. When used wisely and with clear understanding between the classes involved, upcasting can be a great aid in developing well written and maintainable code.
More Stories
The Hidden Tech Talents of Women: How Unique Skills Are Shaping the Future of Coding
How AI Web Scraping is Transforming Data Collection
Future AI Art Trends Shaping Video Creation